الدورات
title
Top 40+ OOPs Interview Questions with Answers for 2025
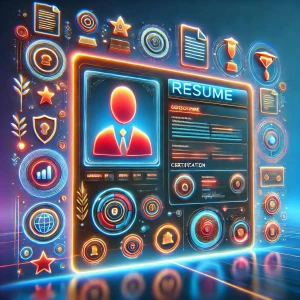
Object-Oriented Programming (OOP) is a fundamental concept in software development, offering features like Encapsulation, Inheritance, Polymorphism, and Abstraction. These four pillars form the foundation of OOP and are critical to understand, especially for technical interviews. Failing to grasp these concepts could cost you opportunities at top tech companies like Microsoft, Google, or Adobe.
To help you prepare, we’ve compiled a list of 40+ OOPs interview questions along with detailed answers. These questions are commonly asked in interviews, and mastering them will boost your confidence and performance.
Basic OOPs Concepts
- What is OOP?
- OOP stands for Object-Oriented Programming. It is a programming paradigm that organizes software design around objects and classes, making it easier to manage and scale complex systems.
- What are the four pillars of OOP?
- The four pillars of OOP are:
- Inheritance: Allows a class to inherit properties and methods from another class.
- Encapsulation: Bundles data and methods into a single unit and restricts access to some components.
- Polymorphism: Enables objects to take on multiple forms (e.g., method overloading and overriding).
- Abstraction: Hides complex implementation details and exposes only essential features.
- What is a class?
- A class is a blueprint or template for creating objects. It defines the properties (attributes) and behaviors (methods) that the objects created from the class will have.
- What is an object?
- An object is an instance of a class. It represents a real-world entity with a state (data) and behavior (methods).
- What is a constructor?
- A constructor is a special method in a class that is automatically called when an object is created. It initializes the object’s state.
- Example in Java:
- java
- Copy
public class MyClass { // Constructor MyClass() { System.out.println("Object created!"); } }
- What are the types of constructors?
- Default Constructor: Automatically created if no constructor is defined.
- Parameterized Constructor: Accepts parameters to initialize objects with specific values.
- Copy Constructor: Creates an object by copying data from another object.
- What is inheritance?
- Inheritance allows a class (child class) to inherit properties and methods from another class (parent class). It promotes code reusability and hierarchical classification.
- What are the types of inheritance?
- Single Inheritance: A class inherits from one parent class.
- Multilevel Inheritance: A class inherits from a derived class, forming a chain.
- Multiple Inheritance: A class inherits from more than one parent class (not supported in Java).
- Hierarchical Inheritance: Multiple classes inherit from a single parent class.
- Hybrid Inheritance: A combination of two or more types of inheritance.
- What is polymorphism?
- Polymorphism allows objects to take on multiple forms. It can be achieved through:
- Compile-time Polymorphism: Method overloading.
- Runtime Polymorphism: Method overriding.
- What is method overloading?
- Method overloading allows a class to have multiple methods with the same name but different parameters. It is an example of compile-time polymorphism.
- What is method overriding?
- Method overriding occurs when a subclass provides a specific implementation of a method already defined in its parent class. It is an example of runtime polymorphism.
- What is encapsulation?
- Encapsulation is the process of bundling data (attributes) and methods (functions) into a single unit (class) and restricting access to some components using access specifiers (e.g., private, protected).
- What is abstraction?
- Abstraction hides complex implementation details and exposes only essential features to the user. It can be achieved using abstract classes and interfaces.
- What is an abstract class?
- An abstract class is a class that cannot be instantiated and may contain abstract methods (methods without implementation). It is used as a base for other classes.
- What is an interface?
- An interface is a reference type in Java that contains abstract methods. It defines a contract for classes to implement, enabling multiple inheritance.
- What are access specifiers?
- Access specifiers define the visibility of classes, methods, and variables. The main types are:
- Public: Accessible from anywhere.
- Private: Accessible only within the class.
- Protected: Accessible within the class and its subclasses.
- Default: Accessible within the same package.
- What is the difference between a class and an object?
- A class is a blueprint, while an object is an instance of a class.
- A class does not occupy memory, whereas an object does.
- What is the difference between method overloading and method overriding?
- Overloading: Same method name, different parameters (compile-time polymorphism).
- Overriding: Same method name and parameters in a subclass (runtime polymorphism).
- What is the difference between abstraction and encapsulation?
- Abstraction: Focuses on hiding complexity and exposing only essential features.
- Encapsulation: Focuses on bundling data and methods into a single unit and restricting access.
- What is the difference between a superclass and a subclass?
- A superclass is the parent class from which properties and methods are inherited.
- A subclass is the child class that inherits from the superclass.
Advanced OOPs Concepts
- What is static and dynamic polymorphism?
- Static Polymorphism: Achieved through method overloading (compile-time).
- Dynamic Polymorphism: Achieved through method overriding (runtime).
- What is a singleton class?
- A singleton class allows only one instance of itself to be created and provides a global point of access to that instance.
- What is the difference between a class and a structure?
- Class: Reference type, supports inheritance, and has access specifiers.
- Structure: Value type, does not support inheritance, and is typically used for lightweight objects.
- What is the difference between multiple and multilevel inheritance?
- Multiple Inheritance: A class inherits from more than one parent class.
- Multilevel Inheritance: A class inherits from a derived class, forming a chain.
- What is the difference between an abstract class and an interface?
- Abstract Class: Can have both abstract and concrete methods, supports constructors, and cannot support multiple inheritance.
- Interface: Contains only abstract methods (in Java 8+, default and static methods are allowed), and supports multiple inheritance.
- What is the "super" keyword?
- The
super
keyword is used to refer to the parent class’s properties, methods, or constructor. - What is the "this" keyword?
- The
this
keyword refers to the current instance of the class and is used to access its members. - What is a final class?
- A final class cannot be inherited or extended by other classes.
- What is a final method?
- A final method cannot be overridden by subclasses.
- What is a final variable?
- A final variable cannot be reassigned after initialization.
- What is a static method?
- A static method belongs to the class rather than an instance and can be called without creating an object.
- What is a static variable?
- A static variable is shared among all instances of a class and retains its value throughout the program’s execution.
- What is a package in Java?
- A package is a namespace that organizes related classes and interfaces.
- What is the difference between composition and inheritance?
- Composition: A class contains objects of other classes (has-a relationship).
- Inheritance: A class inherits properties and methods from another class (is-a relationship).
- What is a virtual function?
- A virtual function is a function in a base class that can be overridden in a derived class. It enables runtime polymorphism.
- What is a pure virtual function?
- A pure virtual function is a function declared in a base class but has no implementation. It makes the class abstract.
- What is a friend function?
- A friend function is a function that is not a member of a class but has access to its private and protected members.
- What is operator overloading?
- Operator overloading allows operators (e.g., +, -, *) to have different implementations based on the operands.
- What is a destructor?
- A destructor is a special method that is automatically called when an object is destroyed. It is used to release resources.
- What is the difference between shallow copy and deep copy?
- Shallow Copy: Copies only the references, not the actual objects.
- Deep Copy: Creates a new copy of the objects and their references.
Conclusion
Mastering these OOPs concepts and interview questions will prepare you for technical interviews and help you stand out as a strong candidate. Practice coding examples and understand the underlying principles to ace your next interview!