الدورات
title
A Clean Code Guide for Front-End Development: Professionalism, Readability, and Mastery
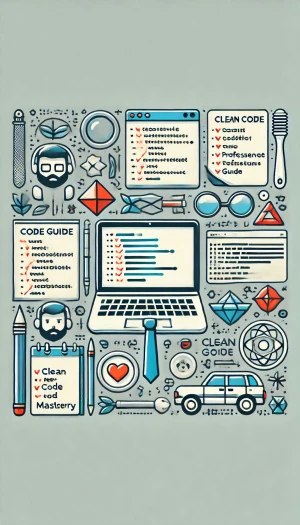
Clean code is more than just an aesthetic goal—it's a foundation for creating maintainable, scalable, and professional-grade software. Drawing inspiration from "The Clean Coder: A Code of Conduct for Professional Programmers" by Robert C. Martin (Uncle Bob), this guide provides actionable tips to help front-end developers write cleaner, more effective code while fostering a culture of professionalism and growth.
1. Professionalism: Discipline in Coding
Professionalism is at the core of The Clean Coder, encouraging developers to take ownership of their work.
1.1. Responsibility for Code
- Own Your Work: If bugs or errors arise, address them promptly and use the experience to learn and improve.
- Seek Clarity: When requirements are unclear, don't proceed on assumptions. Collaborate with stakeholders to ensure a shared understanding.
1.2. Craftsmanship
Approach coding as an art form. Strive to deliver work that balances performance, readability, and maintainability.
2. Code Readability: Clear and Understandable Code
Clean code should read like well-written prose. Aim for simplicity and clarity.
2.1. Favor Simplicity Over Cleverness
Clever code can be impressive but often hinders collaboration.
Example:
Instead of:
const d = (x) => x % 2 ? 'odd' : 'even'; // Confusing
Write:
const getOddOrEven = (number) => number % 2 === 0 ? 'even' : 'odd'; // Clear and understandable
2.2. Meaningful Names
Use descriptive variable and function names that convey intent.
- Functions: Use verbs (e.g.,
fetchMovies
,calculateTotal
). - Variables: Use nouns (e.g.,
userList
,movieDetails
).
2.3. Small, Focused Functions
Keep functions concise and focused on a single task. Limit them to 5-10 lines of code whenever possible.
Example:
Bad:
function renderMovieCard(movie) { // Fetch data, format, and render all in one function }
Good:
Break it into smaller, reusable functions like fetchMovieDetails
, formatMovieData
, and renderMovieCard
.
3. Separation of Concerns
Each part of your code should focus on one responsibility.
3.1. Divide Responsibilities
- Components: Handle rendering and UI.
- Logic: Encapsulate in hooks or utility functions.
- Styles: Use CSS Modules, utility-first CSS (e.g., Tailwind), or styled-components.
3.2. Example: Clean Separation in a React Component
Bad:
function MovieCard({ movie }) { const isBlockbuster = movie.revenue > 1000000; return{movie.title}; }
Good:
// hooks/useIsBlockbuster.js export const useIsBlockbuster = (revenue) => revenue > 1000000; // components/MovieCard.js import { useIsBlockbuster } from '../hooks/useIsBlockbuster'; import styles from './MovieCard.module.css'; function MovieCard({ movie }) { const isBlockbuster = useIsBlockbuster(movie.revenue); return{movie.title}; }
4. Self-Documenting Code
Strive for code that explains itself, reducing the need for external documentation.
4.1. Abstract Intent
Replace "magic numbers" with named constants and unclear logic with well-named variables.
Before:
if (user.role === 1) { // Admin-specific logic }
After:
const isAdmin = user.role === USER_ROLES.ADMIN; if (isAdmin) { // Admin-specific logic }
5. Testing: The Safety Net
Testing ensures your code works as intended and minimizes bugs.
5.1. Coverage Goals
- Aim for 80% unit test coverage.
- Include integration and end-to-end (E2E) tests for key user workflows.
5.2. Write Meaningful Tests
Tests should verify behavior and requirements, not implementation.
Example Test:
test('renders movie title on card', () => { render(); expect(screen.getByText('Inception')).toBeInTheDocument(); });
6. Continuous Improvement
Adopt a mindset of perpetual growth and refinement.
6.1. Refactor Regularly
Tackle tech debt and improve existing code whenever possible. Follow the Boy Scout Rule: Leave the codebase cleaner than you found it.
6.2. Embrace New Tools
Stay updated with advancements like React Server Components, AI-assisted UX, or modern state management libraries (e.g., Redux Toolkit, Zustand).
7. Time Management and Collaboration
7.1. Work in Iterations
Break tasks into smaller deliverables to maintain focus and consistency.
7.2. Communicate Clearly
Regular updates, effective code reviews, and proactive issue discussions foster better teamwork.
8. Clean Architecture Principles for Front-End Development
8.1. Separate UI and State Management
Centralize complex state management with libraries like Redux Toolkit, while keeping simple state local to components.
8.2. Dependency Injection
Pass dependencies (e.g., APIs or services) as props to enhance testability and flexibility.
9. Never Stop Learning
Clean code is a lifelong pursuit. Invest in books like "Clean Code", "Clean Architecture", or "Refactoring". Contribute to open source or build side projects to practice and refine your skills.